mirror of
https://github.com/picocss/pico.git
synced 2025-07-06 12:52:38 -04:00
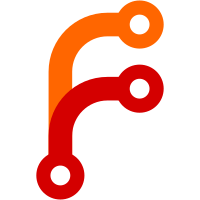
Added cursor back on button - it was just an arrow. Added parent-selector to the notifications, and corrected readbility of notifications. updated the version within the css files made rows only show up on css files where classes are enabled made the tooltips have a dark background - even for dark themes.
101 lines
2.9 KiB
SCSS
101 lines
2.9 KiB
SCSS
@use "sass:string";
|
|
@use "sass:map";
|
|
@use "sass:math";
|
|
@use "../settings" as *; // for spacing, breakpoints, and if columns are defined.
|
|
|
|
/* Source inspired by https://github.com/sophie-thomas/CSS-Grid/blob/main/assets/scss/grid.scss */
|
|
|
|
@if map.get($modules, "layout/row") and $enable-classes {
|
|
$helper-cols: "";
|
|
$helper-offset: "";
|
|
/*--- CSS Grid ---*/
|
|
.row-fluid,
|
|
.row {
|
|
display: grid;
|
|
grid-template-columns: repeat($row-columns, 1fr);
|
|
gap: var(#{$css-var-prefix}grid-row-gap) var(#{$css-var-prefix}grid-column-gap);
|
|
&.align-center {
|
|
align-items: center;
|
|
}
|
|
&.align-start {
|
|
align-items: start;
|
|
}
|
|
&.align-end {
|
|
align-items: end;
|
|
}
|
|
> [class*="col"] > *,
|
|
> [class|="col"] > *,
|
|
> [class~="col"] > * {
|
|
margin: calc(var(#{$css-var-prefix}block-spacing-vertical) * 0.5) auto;
|
|
}
|
|
}
|
|
.row {
|
|
max-width: map.get(map.get($breakpoints, "xl"), "viewport");
|
|
margin: 0 auto;
|
|
}
|
|
/* Defining columns spans and offsets */
|
|
// Loop through all column spans and define the .grid-column-end number
|
|
@for $col from 1 through $row-columns {
|
|
.col-#{$col} {
|
|
grid-column-end: span $col;
|
|
}
|
|
@if $col == 1 {
|
|
$helper-cols: ".col-1";
|
|
} @else {
|
|
$helper-cols: #{$helper-cols} + ", .col-" + #{$col};
|
|
}
|
|
}
|
|
|
|
// Loop through all column offsets and define the .grid-column-start number
|
|
@for $offset from 0 through $row-columns - 1 {
|
|
.offset-#{$offset} {
|
|
grid-column-start: $offset + 1;
|
|
}
|
|
@if $offset == 0 {
|
|
$helper-offset: ".offset-0";
|
|
} @else {
|
|
$helper-offset: #{$helper-offset} + ", .offset-" + #{$offset};
|
|
}
|
|
}
|
|
// Defines media queries for each breakpoint and loops through both spans
|
|
// and offsets to set grid-column-end and grid-column-start
|
|
// Loop through breakpoints and generate media queries
|
|
|
|
@each $breakpoint, $values in $breakpoints {
|
|
@if $values {
|
|
@media (min-width: map.get($values, "viewport")) {
|
|
@for $col from 1 through $row-columns {
|
|
.col-#{$breakpoint}-#{$col} {
|
|
grid-column-end: span $col;
|
|
}
|
|
@if ($breakpoint != "sm") {
|
|
$helper-cols: #{$helper-cols} + ", .col-" + #{$breakpoint} + "-" + #{$col};
|
|
}
|
|
}
|
|
@for $offset from 0 through $row-columns - 1 {
|
|
.offset-#{$breakpoint}-#{$offset} {
|
|
grid-column-start: $offset + 1;
|
|
}
|
|
@if ($breakpoint != "sm") {
|
|
$helper-offset: #{$helper-offset} + ", .offset-" + #{$breakpoint} + "-" + #{$offset};
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
/* CSS Grid Media Queries */
|
|
// Sets the columns to be col-12 with a starting column of 1
|
|
$sm-size: map.get(map.get($breakpoints, "sm"), "viewport");
|
|
|
|
@media (max-width: $sm-size) {
|
|
//[class*="col-"] {
|
|
#{$helper-cols} {
|
|
grid-column-end: span $row-columns;
|
|
}
|
|
//[class*="offset-"] {
|
|
#{$helper-offset} {
|
|
grid-column-start: 1;
|
|
}
|
|
}
|
|
}
|