mirror of
https://github.com/CorentinTh/it-tools.git
synced 2025-04-25 09:16:15 -04:00
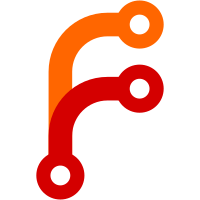
* #521 Random MAC address generator * refactor(mac-address-generator): improved ux * refactor(mac-address-generator): improved ux --------- Co-authored-by: Corentin THOMASSET <corentin.thomasset74@gmail.com>
18 lines
722 B
TypeScript
18 lines
722 B
TypeScript
import _ from 'lodash';
|
|
|
|
export { splitPrefix, generateRandomMacAddress };
|
|
|
|
function splitPrefix(prefix: string): string[] {
|
|
const base = prefix.match(/[^0-9a-f]/i) === null ? prefix.match(/.{1,2}/g) ?? [] : prefix.split(/[^0-9a-f]/i);
|
|
|
|
return base.filter(Boolean).map(byte => byte.padStart(2, '0'));
|
|
}
|
|
|
|
function generateRandomMacAddress({ prefix: rawPrefix = '', separator = ':', getRandomByte = () => _.random(0, 255).toString(16).padStart(2, '0') }: { prefix?: string; separator?: string; getRandomByte?: () => string } = {}) {
|
|
const prefix = splitPrefix(rawPrefix);
|
|
|
|
const randomBytes = _.times(6 - prefix.length, getRandomByte);
|
|
const bytes = [...prefix, ...randomBytes];
|
|
|
|
return bytes.join(separator);
|
|
}
|