mirror of
https://github.com/CorentinTh/it-tools.git
synced 2025-04-22 15:56:15 -04:00
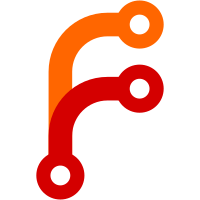
* feat(new tool): Regex Tester Fix https://github.com/CorentinTh/it-tools/issues/1007, https://github.com/CorentinTh/it-tools/issues/991, https://github.com/CorentinTh/it-tools/issues/936, https://github.com/CorentinTh/it-tools/issues/761, https://github.com/CorentinTh/it-tools/issues/649 https://github.com/CorentinTh/it-tools/issues/644, https://github.com/CorentinTh/it-tools/issues/554 https://github.com/CorentinTh/it-tools/issues/308 * fix: refactor to service + add regex diagram + ui enhancements * fix: update queryParams * fix: deps * fix: svg style bug in @regexper/render @regexper/render use a stylesheet in svg that cause bugs in whole site. So add regexper in a shadow root * feat(new tool): added Regex Cheatsheet * Update src/tools/regex-memo/index.ts * Update src/tools/regex-tester/index.ts --------- Co-authored-by: Corentin THOMASSET <corentin.thomasset74@gmail.com>
61 lines
1.6 KiB
TypeScript
61 lines
1.6 KiB
TypeScript
interface RegExpGroupIndices {
|
|
[name: string]: [number, number]
|
|
}
|
|
interface RegExpIndices extends Array<[number, number]> {
|
|
groups: RegExpGroupIndices
|
|
}
|
|
interface RegExpExecArrayWithIndices extends RegExpExecArray {
|
|
indices: RegExpIndices
|
|
}
|
|
interface GroupCapture {
|
|
name: string
|
|
value: string
|
|
start: number
|
|
end: number
|
|
};
|
|
|
|
export function matchRegex(regex: string, text: string, flags: string) {
|
|
// if (regex === '' || text === '') {
|
|
// return [];
|
|
// }
|
|
|
|
let lastIndex = -1;
|
|
const re = new RegExp(regex, flags);
|
|
const results = [];
|
|
let match = re.exec(text) as RegExpExecArrayWithIndices;
|
|
while (match !== null) {
|
|
if (re.lastIndex === lastIndex || match[0] === '') {
|
|
break;
|
|
}
|
|
const indices = match.indices;
|
|
const captures: Array<GroupCapture> = [];
|
|
Object.entries(match).forEach(([captureName, captureValue]) => {
|
|
if (captureName !== '0' && captureName.match(/\d+/)) {
|
|
captures.push({
|
|
name: captureName,
|
|
value: captureValue,
|
|
start: indices[Number(captureName)][0],
|
|
end: indices[Number(captureName)][1],
|
|
});
|
|
}
|
|
});
|
|
const groups: Array<GroupCapture> = [];
|
|
Object.entries(match.groups || {}).forEach(([groupName, groupValue]) => {
|
|
groups.push({
|
|
name: groupName,
|
|
value: groupValue,
|
|
start: indices.groups[groupName][0],
|
|
end: indices.groups[groupName][1],
|
|
});
|
|
});
|
|
results.push({
|
|
index: match.index,
|
|
value: match[0],
|
|
captures,
|
|
groups,
|
|
});
|
|
lastIndex = re.lastIndex;
|
|
match = re.exec(text) as RegExpExecArrayWithIndices;
|
|
}
|
|
return results;
|
|
}
|