mirror of
https://github.com/ether/etherpad-lite.git
synced 2025-04-24 01:16:15 -04:00
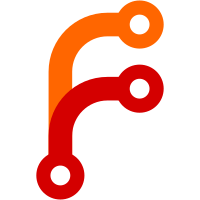
test case uses "nodeify" to convert the calls to TidyHtml back into nodeback because it integrates better with the test framework
43 lines
1.2 KiB
JavaScript
43 lines
1.2 KiB
JavaScript
/**
|
|
* Tidy up the HTML in a given file
|
|
*/
|
|
|
|
var log4js = require('log4js');
|
|
var settings = require('./Settings');
|
|
var spawn = require('child_process').spawn;
|
|
|
|
exports.tidy = function(srcFile) {
|
|
var logger = log4js.getLogger('TidyHtml');
|
|
|
|
return new Promise((resolve, reject) => {
|
|
|
|
// Don't do anything if Tidy hasn't been enabled
|
|
if (!settings.tidyHtml) {
|
|
logger.debug('tidyHtml has not been configured yet, ignoring tidy request');
|
|
return resolve(null);
|
|
}
|
|
|
|
var errMessage = '';
|
|
|
|
// Spawn a new tidy instance that cleans up the file inline
|
|
logger.debug('Tidying ' + srcFile);
|
|
var tidy = spawn(settings.tidyHtml, ['-modify', srcFile]);
|
|
|
|
// Keep track of any error messages
|
|
tidy.stderr.on('data', function (data) {
|
|
errMessage += data.toString();
|
|
});
|
|
|
|
tidy.on('close', function(code) {
|
|
// Tidy returns a 0 when no errors occur and a 1 exit code when
|
|
// the file could be tidied but a few warnings were generated
|
|
if (code === 0 || code === 1) {
|
|
logger.debug('Tidied ' + srcFile + ' successfully');
|
|
resolve(null);
|
|
} else {
|
|
logger.error('Failed to tidy ' + srcFile + '\n' + errMessage);
|
|
reject('Tidy died with exit code ' + code);
|
|
}
|
|
});
|
|
});
|
|
}
|